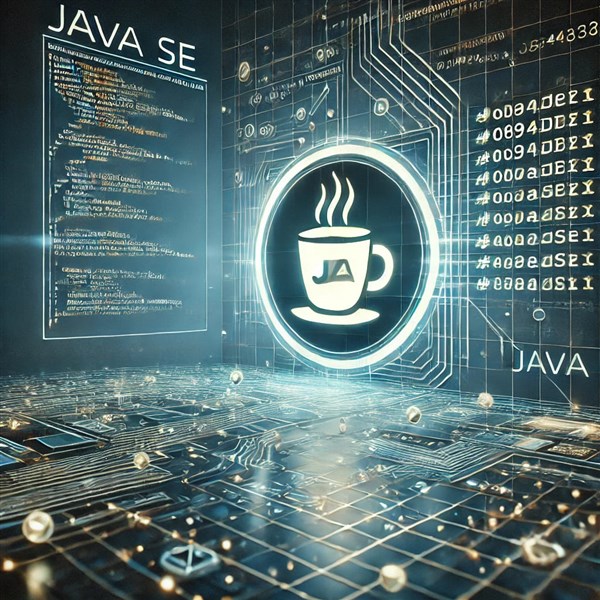
Java Standard Edition (Java SE) is the foundation of the Java programming language, providing core functionalities that power everything from enterprise applications to Android development. Over the years, Java SE has evolved significantly, introducing powerful features that enhance performance, security, and developer productivity.
Whether you're a beginner or an experienced Java developer, understanding these key Java SE features can help you write efficient, scalable, and modern Java applications. In this blog, we’ll explore the top 10 Java SE features every developer should know.
Top 10 Java SE Features Every Developer Should Know
1. Java SE Platform Modules (Introduced in Java 9 - Jigsaw Project)
What It Is:
The Java Platform Module System (JPMS) introduced in Java 9 modularized the JDK, making Java applications more lightweight and efficient.
Why It Matters:
✔ Reduces memory footprint by using only necessary modules
✔ Improves security and performance
✔ Enhances dependency management
Example: Creating a Simple Java Module
java
CopyEdit
module com.example.myapp {
requires java.base; // Declaring dependencies
exports com.example.services; // Making packages accessible
}
💡 Use Case: Modularization is useful for enterprise Java applications, making them scalable and maintainable.
2. Lambda Expressions (Introduced in Java 8)
What It Is:
Lambdas allow you to write concise, functional-style code without defining separate methods or classes.
Why It Matters:
✔ Reduces boilerplate code
✔ Improves readability and maintainability
✔ Enables functional programming in Java
Example: Using a Lambda in Java
java
CopyEdit
// Traditional Anonymous Class
Runnable oldWay = new Runnable() {
public void run() {
System.out.println("Running...");
}
};
// Lambda Expression
Runnable newWay = () -> System.out.println("Running...");
💡 Use Case: Lambdas simplify handling event-driven programming and stream processing.
3. Streams API (Introduced in Java 8)
What It Is:
The Streams API provides a functional way to process collections, making data manipulation more readable and efficient.
Why It Matters:
✔ Simplifies filtering, mapping, and reducing data
✔ Improves code performance with parallel processing
✔ Encourages declarative programming
Example: Filtering a List Using Streams
java
CopyEdit
import java.util.List;
import java.util.stream.Collectors;
List<String> names = List.of("John", "Alice", "Bob", "Jane");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [John, Jane]
💡 Use Case: Ideal for large-scale data processing in finance and analytics applications.
4. Records (Introduced in Java 14)
What It Is:
Records allow you to define immutable data objects with minimal boilerplate.
Why It Matters:
✔ Reduces code complexity
✔ Improves readability
✔ Supports data encapsulation
Example: Defining a Record
java
CopyEdit
public record Person(String name, int age) {}
Person p = new Person("Alice", 30);
System.out.println(p.name()); // Alice
💡 Use Case: Perfect for DTOs (Data Transfer Objects) in REST APIs.
5. Pattern Matching (Introduced in Java 16 & 17)
What It Is:
Pattern Matching simplifies type checking and casting, reducing redundancy.
Why It Matters:
✔ Reduces boilerplate code in conditionals
✔ Improves type safety
Example: Pattern Matching in instanceof
java
CopyEdit
Object obj = "Hello, Java";
if (obj instanceof String s) {
System.out.println(s.toUpperCase()); // HELLO, JAVA
}
💡 Use Case: Useful in data validation and object processing.
6. Sealed Classes (Introduced in Java 17)
What It Is:
Sealed classes restrict which classes can extend them, providing better control over inheritance.
Why It Matters:
✔ Improves security and encapsulation
✔ Enhances API maintainability
Example: Defining a Sealed Class
java
CopyEdit
sealed class Vehicle permits Car, Truck {}
final class Car extends Vehicle {}
final class Truck extends Vehicle {}
💡 Use Case: Used in domain-driven design (DDD) and API security.
7. Multithreading and Concurrency Enhancements
What It Is:
Java SE provides powerful concurrency utilities for parallel processing.
Why It Matters:
✔ Supports multi-core processing
✔ Reduces execution time
Example: Using ExecutorService
java
CopyEdit
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
ExecutorService executor = Executors.newFixedThreadPool(3);
executor.execute(() -> System.out.println("Task 1 executed"));
executor.shutdown();
💡 Use Case: Critical for high-performance web applications.
8. Improved Garbage Collection (G1 & ZGC)
What It Is:
Java SE introduced G1 and ZGC collectors for better memory management.
Why It Matters:
✔ Reduces pause times
✔ Improves performance for large heap sizes
Example: Enabling ZGC
shell
CopyEdit
java -XX:+UseZGC MyApplication
💡 Use Case: Useful for high-memory applications like data analytics.
9. Enhanced Switch Expressions (Introduced in Java 14)
What It Is:
Improved switch expressions provide concise syntax and better control flow.
Example: Using Switch Expression
java
CopyEdit
String result = switch (day) {
case "Monday", "Tuesday" -> "Weekday";
case "Saturday", "Sunday" -> "Weekend";
default -> "Unknown";
};
💡 Use Case: Ideal for state management in applications.
10. Java Flight Recorder & Mission Control
What It Is:
Java SE includes Java Flight Recorder (JFR) for performance monitoring.
Why It Matters:
✔ Helps identify performance bottlenecks
✔ Provides real-time system insights
💡 Use Case: Used in performance tuning for Java applications.
Final Thoughts
Mastering these top 10 Java SE features will help you write efficient, scalable, and modern Java applications. Whether you're a web developer, backend engineer, or cloud architect, these features are essential for Java programming success.
💡 Which Java SE feature do you use most? Let us know in the comments! 🚀
At Koenig Solutions, we provide world-class training on these features and more through our comprehensive Java SE training and certification courses. Our courses are designed by industry experts and are aimed at helping you master the latest Java SE features to enhance your development skills and career prospects.
COMMENT